Before using FastAPI you’ll need to install a few Python packages. First is the fastapi
package. As the name suggests this contains the FastAPI framework modules. Next, you’ll need uvicorn
. This is an ASGI server that will run the FastAPI application. Use pip
to install both.
$ pip install fastapi uvicorn
In a new Python file (I’ll use the name main.py
) import the FastAPI
class from the fastapi
module.
from fastapi import FastAPI
Next, create a new instance of the FastAPI
class. I’ll use the variable api
.
api = FastAPI()
To map URLs to handler functions, apply a decorator to a function that will handle requests to the URL. Here the get
decorator maps the root URL to the hello_world
function.
The hello_world
function simply returns a Python dictionary. Since FastAPI is designed for REST APIs, you don’t need to render a template like you would with Django or Flask. A REST API is likely going to respond with JSON data. Conveniently, Python dictionaries and list transform nicely into JSON objects and arrays. FastAPI will transform Python types to JSON when possible.
@api.get("/")
def hello_world():
return {"message": "Hello FastAPI")
Here is the complete code for the main.py
file:
from fastapi import FastAPI
api = FastAPI()
@api.get("/")
def hello_world():
return {"message": "Hello FastAPI"}
To run the application invoke uvicorn
from the command line. It expects the name of the FastAPI instance which here is api
inside of the main
module. The —reload
option will watch for changes in the code and restart if needed.
$ uvicorn main:api --reload
As with all REST APIs, you could use a tool like Postman to test the application. However, FastAPI includes a web interface that makes ad hoc testing much easier. The interface can be found at /docs
.
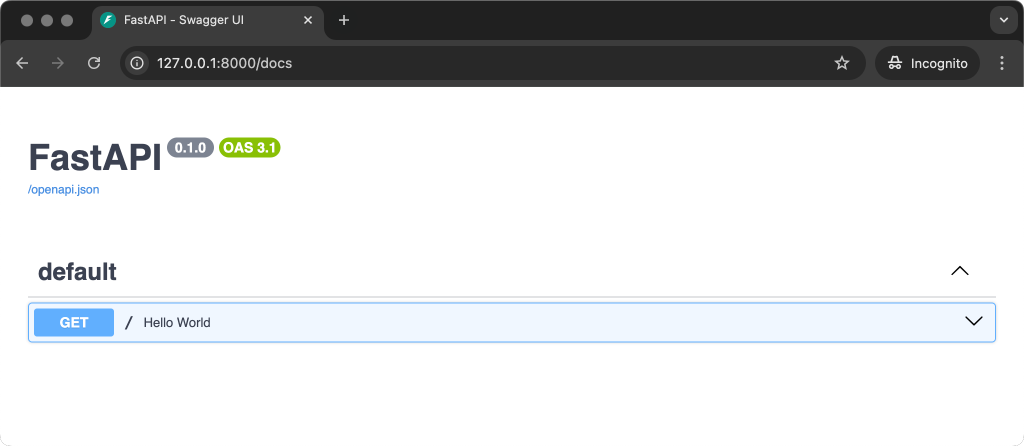
To interact with an endpoint expand an item.
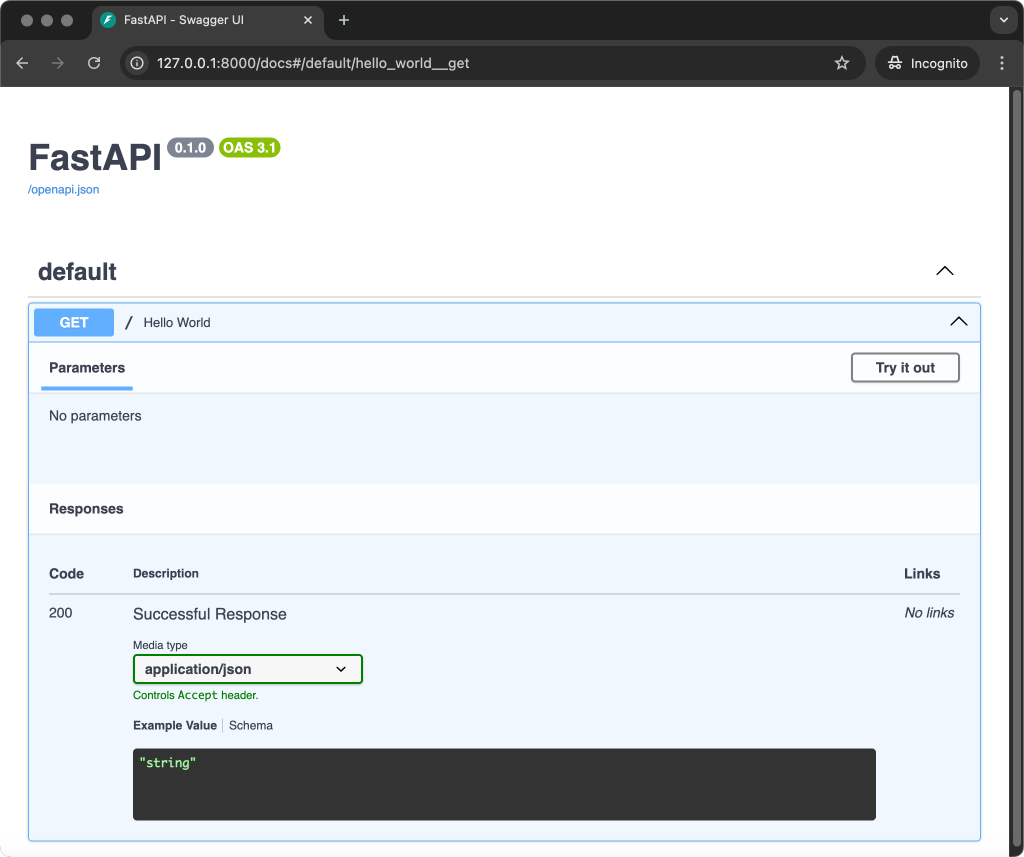
Click the Try it out button to configure an HTTP request. There are no options for this endpoint so click the blue Execute button.
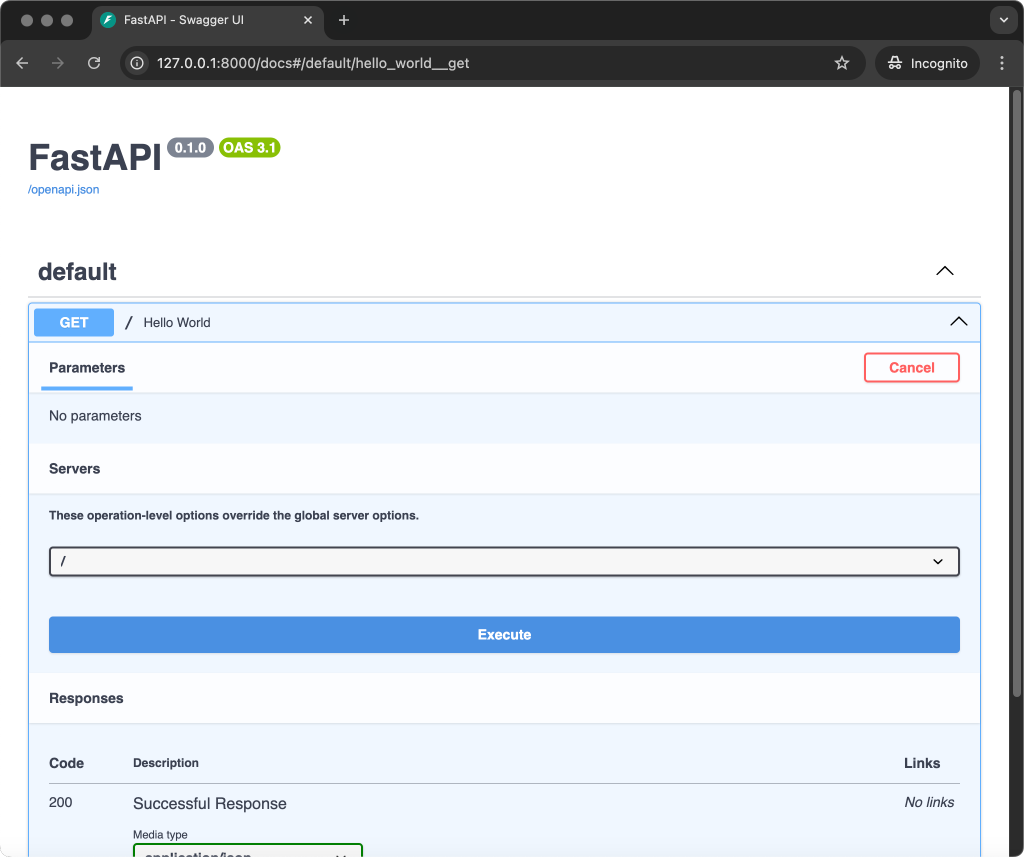
Scroll down a little and inspect the response. As expected, the body of the response includes the JSON data returned by the hello_world
function.
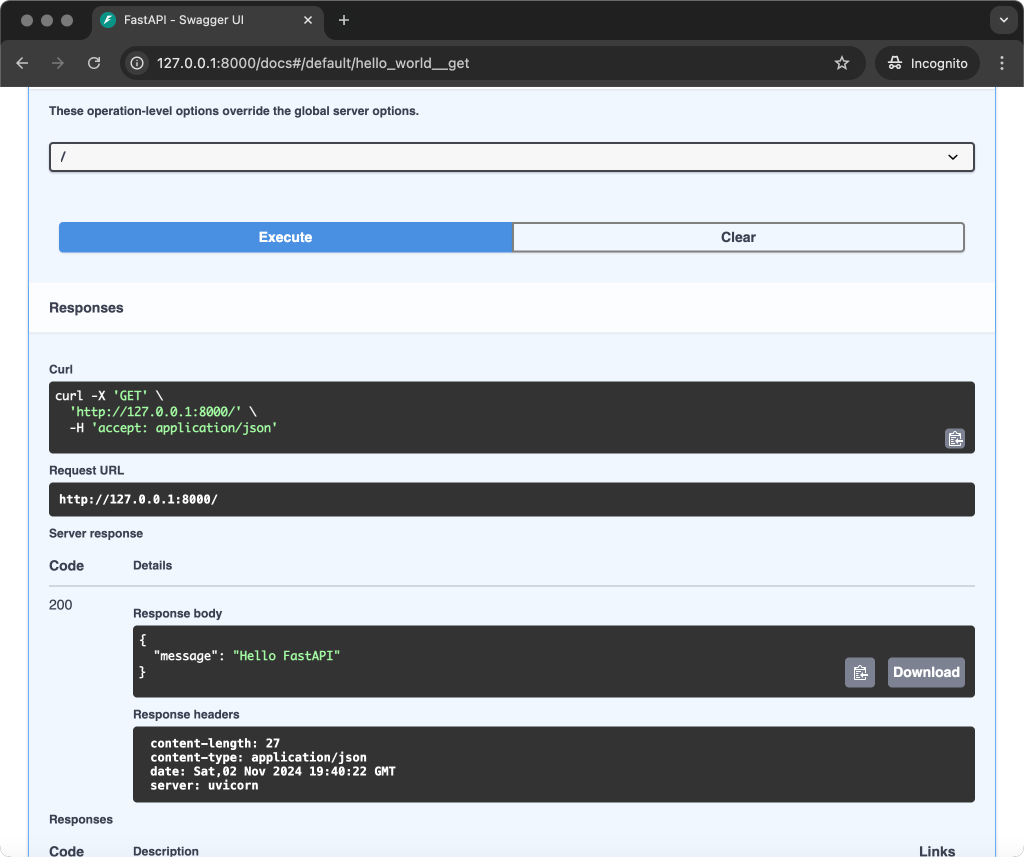
That’s how easy it is to get started!